Introducing Ionic Animations
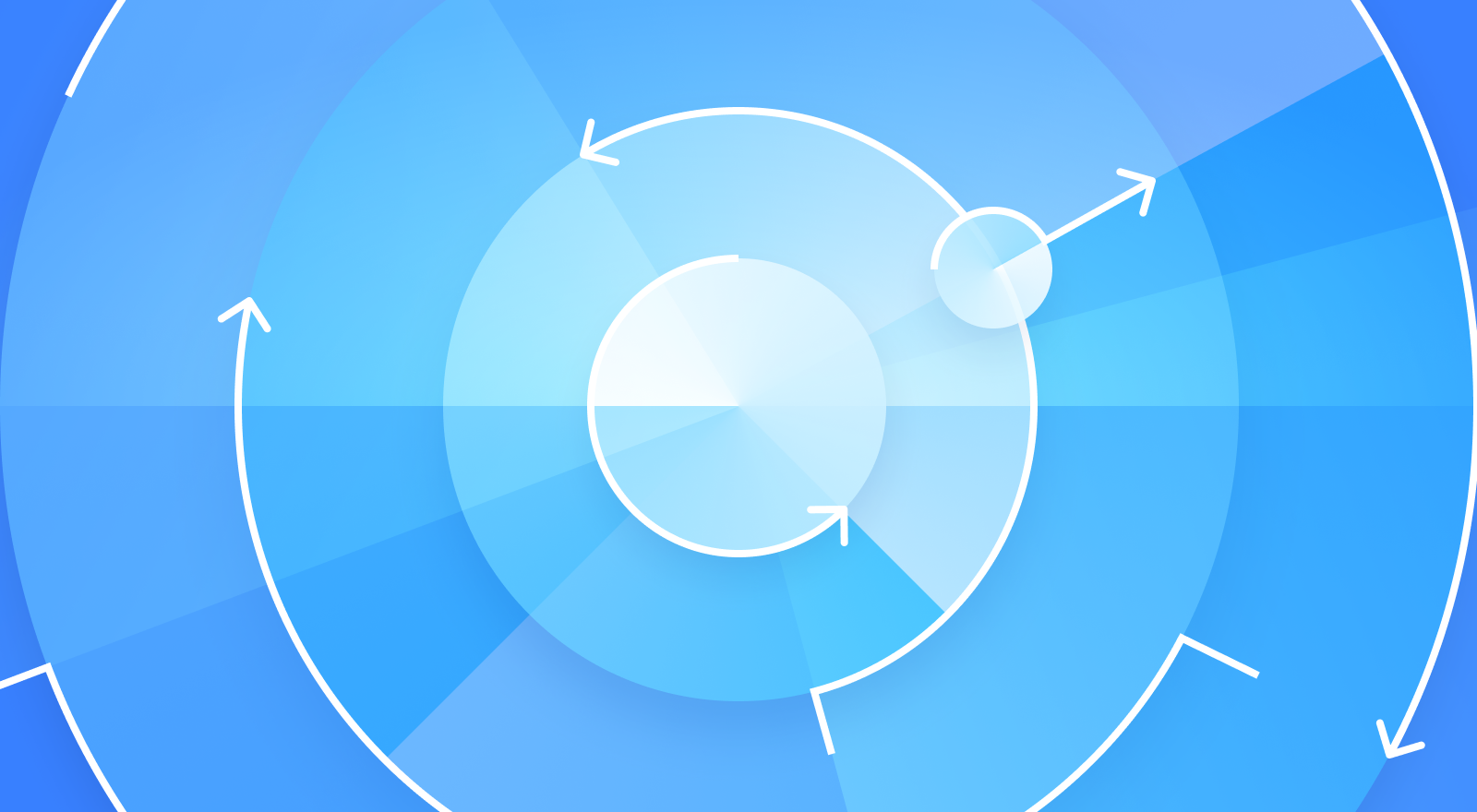
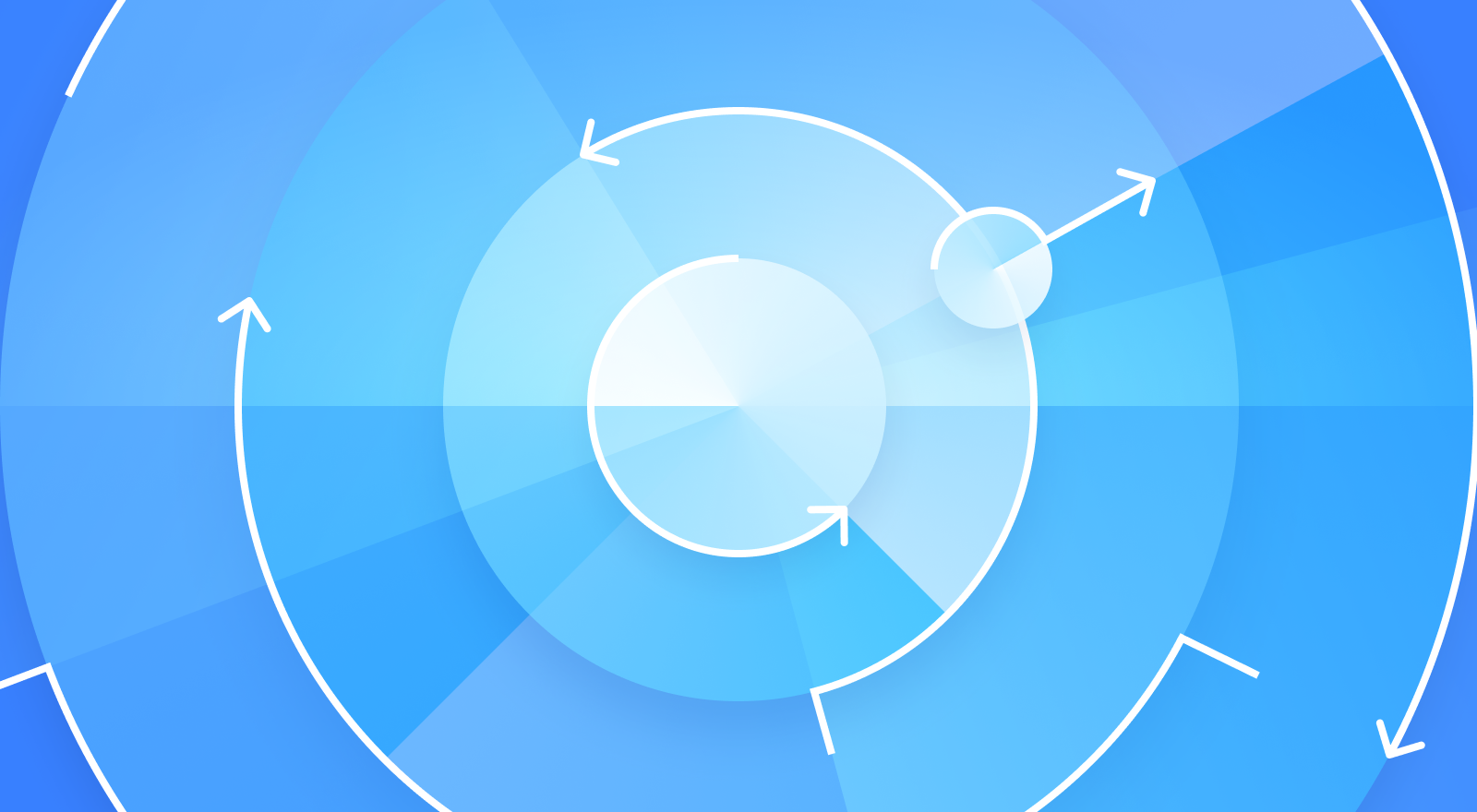
Building efficient animations has traditionally been hard. Developers are often limited by the libraries available to them as well as the hardware that their apps run on. On top of that, many of these animation libraries use a JavaScript-driven approach to running animations where they handle the calculation of your animation’s values at every step in a requestAnimationFrame
event loop.
To combat these issues we have created Ionic Animations. Ionic Animations is an open source animations utility that provides the tools developers need to build highly performant animations regardless of the framework they are using. Ionic Animations is more performant than leading animation libraries, which is why we are especially excited about its release. Officially part of our upcoming 5.0 release, we wanted to take some time to dig into what Ionic Animations is and why it is different than other animation libraries.
Why Ionic Animations?
As mentioned previously, many animation libraries use a JavaScript-driven approach to running your animations. This approach is problematic as not only is the browser rendering your animation, it is also executing your animation event loop code at every single frame in the same thread as the rest of your application’s logic. By forcing the browser to execute your code on every frame, valuable CPU time is being used that will ultimately impact overall frame rate and energy consumption. While overall frames per second (FPS) is important, it is also crucial to understand the impact your animation is having on the device’s resources.
Ionic Animations uses the Web Animations API to build and run all of your animations. By doing this, we can offload all of the work required to run your animations to the browser. As a result, the browser is able to schedule and make any optimizations to your animations that it needs, allowing your animations to run as smoothly as possible. This leads to high FPS while maintaining low CPU usage and therefore a lower energy impact. While most modern browsers support Web Animations, we fallback to CSS Animations for browsers that do not. In these instances, the performance difference in switching between the two should typically be negligible.
Ionic Animations
The Ionic Animations API is straight forward and allows developers to create grouped animations, gesture animations, and much more. It currently powers all animations in Ionic Framework including the gesture animations for the menu and swipe to go back.
Since we are using native web APIs, all animations created naturally have a low CPU and battery usage overhead, allowing your animations to take full advantage of the processing resources available to them.
Let’s take a look at some examples.
Ionic Animations provides a chainable API that lets you declare your animation properties with ease. In the example below we’ve created a pulsating effect by animating the scale and changing the direction of the animation on each iteration via the direction
method.
const animation = createAnimation()
.addElement(document.querySelector('.square'))
.duration(1000)
.direction('alternate')
.iterations(Infinity)
.keyframes([
{ offset: 0, transform: 'scale(1)', opacity: '1' },
{ offset: 1, transform: 'scale(1.5)', opacity: '0.5'
}
]);
animation.play();
You can also group animations and control them using a single parent animation. This gives you the ability to control complex animations with different durations, easings, and other properties.
const animationA = createAnimation()
.addElement(document.querySelector('.square-a'))
.fromTo('transform', 'scale(1)', 'scale(1.5)');
const animationB = createAnimation()
.addElement(document.querySelector('.square-b'))
.fromTo('transform', 'scale(1)', 'scale(0.5)');
const parentAnimation = createAnimation()
.duration(1000)
.addAnimation([animationA, animationB]);
parentAnimation.play();
Chaining animations is as simple as awaiting a promise!
const animationA = createAnimation()
.addElement(document.querySelector('.square-a'))
.duration(1000)
.fromTo('transform', 'scale(1)', 'scale(1.5)');
const animationB = createAnimation()
.addElement(document.querySelector('.square-b'))
.duration(2000)
.fromTo('transform', 'scale(1)', 'scale(0.5)');
await animationA.play();
await animationB.play();
Ionic Animations also gives you the ability to control animation playback and provides hooks to perform DOM reads and writes, as well as add or remove classes and inline styles.
const animation = createAnimation()
.addElement(document.querySelector('.square'))
.duration(1000)
.fromTo('transform', 'scale(1)', 'scale(1.5)');
.afterStyles({
'background': 'green'
})
await animation.play();
Performance Comparisons
When developing Ionic Animations we performed a number of tests to ensure that it was living up to our expectations in terms of performance and resource consumption. We also looked at other animation libraries such as anime.js, GSAP, and Velocity.js to see how Ionic Animations compares to some of the leading alternatives.
We developed a simple test to see how these different libraries performed when animating a large number of elements. We ran this test on both a desktop device as well as a mobile device to get a sense of performance when dealing with different hardware constraints. The test we built animates a variable number of bubbles floating up the screen at different rates of speed.
We developed the following performance benchmarks to look at:
- Average FPS — This is the average frames per second over the course of the animation test. This is typically the benchmark that end users will notice the most, so it’s important that this is as close to 60fps as possible.
-
Main Thread Processing — This is the amount of work that the browser needs to do as a result of your application’s code. This includes things such as layouts, paints, and computing JavaScript. All of this happens in the same thread as the rest of your application’s logic.
-
Average CPU Usage — This is the average percentage of the CPU that is actively working during the test as a result of your code. While using the CPU is inevitable, it is important to minimize the overall CPU usage and ensure that the usage goes back to idle as soon as possible.
-
Energy Impact — This is the effect the animation has on energy consumption. Animations with a higher energy impact will drain a device’s battery faster than animations with a lower energy impact.
This blog post will show a subset of all data collected. You can view the full report here. The desktop test shows Ionic Animations using the Web Animations API in Safari while the mobile test shows Ionic Animations using the CSS Animations fallback. You can also run these tests for yourself on the demo site that we have setup: flamboyant-brattain-9c21f4.netlify.com
Desktop Animation Benchmarks
This first test looks at the Ionic Animations performance relative to other libraries on a desktop machine. [1]
Frames per Second (FPS)
Higher is better
Average CPU Usage
Lower is better
The data above shows Ionic Animations easily achieving 60fps over the course of the test while maintaining a low CPU usage. The other libraries had CPU usages of >100% and high energy impacts while only achieving framerates in the 30s and 40s.
On the desktop test, Ionic Animations shows that it can achieve smooth 60fps animations while minimizing not only the amount of work it has to do in the CPU but also the impact it has on your device’s battery.
Mobile Animation Benchmarks
This second test was run on an iPhone 7. We originally wanted to run the same desktop test with 1000 bubbles, but GSAP was unable to run the animation due to a high startup overhead, so we opted to test with 500 bubbles instead. [2]
Frames per Second (FPS)
Higher is better
Average CPU Usage
Lower is better
Similar to the desktop results, Ionic Animations easily achieves 60fps while having a low CPU usage relative to other libraries. The only other library that comes close to matching the Ionic Animations FPS is GSAP, but even then GSAP had to use almost 82% of the CPU to do that while Ionic only needed about 11%.
Once Web Animations ships on iOS you can expect to see an even lower CPU usage for Ionic Animations!
Getting Started and Providing Feedback
As we’ve seen in the tests above, Ionic Animations brings performant animations with a low energy impact to both desktop and mobile devices. We are very excited to have developers start using it.
To get started with Ionic Animations, check out the Ionic Animations Documentation.
We encourage anyone using Ionic Animations to file bugs or feature requests on our GitHub issue tracker.
We hope you enjoy using Ionic Animations!